Journey to an intelligent Azure Chat Bot - Part 5
In this blog series we build a versatile and useful chatbot based on azure, microsoft bot framework, nodejs, teams, chatgpt. Today - Dialog Design, Prompts, Validation and custom API Integrations.
First, I have to mention: As of today, I have no clue yet how to build a chatbot with Microsoft Azure. None of the steps below I have done before - Research will be necessary.
That said - the following are the goals and also the features of the bot built in this blog series. Achieving those goals will certainly facilitate a lot of the daily business:
- Part 1 - Have an Azure Chatbot built in NodeJS
- Part 2 - Deploy the Bot to azure with an automated CICD pipeline
- Part 3 - Integrate the Bot in a Microsoft Teams Channel
- Part 4 - Test how to build multiple choice selections, user intent, dialog flows, prompts and answer validation. Support for Custom API Interactions.
- Part 5 - Test Azure Conversational Language Understanding (CLU)
Part-5 Content
- Introduction
- Conversational Language Understanding
- Language Service Deployment
- Training Language Model
- Bot Implementation
- Conclusion
Introduction
Today we already have part 5—the last part for now - of this blog series. I hope you can learn and benefit as much as I did from the journey, beginning to create our first chat bots based on Microsoft Azure technology. We have already implemented a lot of the useful features.
Let me know if you have other ideas or input about the topic, and I might do some more parts for the blog series.
For research about the topic, I had a look at the following page:
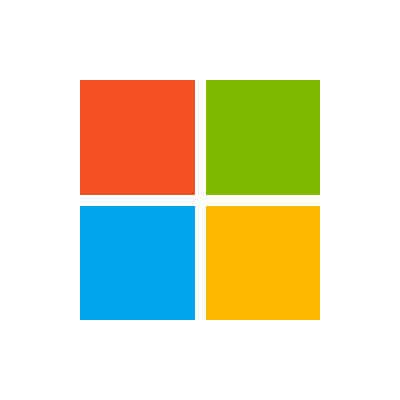
Conversational Language understanding
At this stage, we have still hardcoded a dialog in our bot that gets the user's intent. It asks, "Would you like to order food or beverages?". Then a multiple-choice selection appears: "food" or "beverages".
What we like to achieve now is that the user can enter an arbitrary prompt like: "Can I have a tall glass of iced hibiscus tea?"
The bot should then do language recognition, derive the intent "order beverage" from this prompt, and launch the beverage order dialog.
Language Service Deployment
We will deploy a new language service on Microsoft Azure that does the heavy lifting. It will help create a language model in order to recognize the user's intent. Open your Azure subscription and start provisioning a new language service.
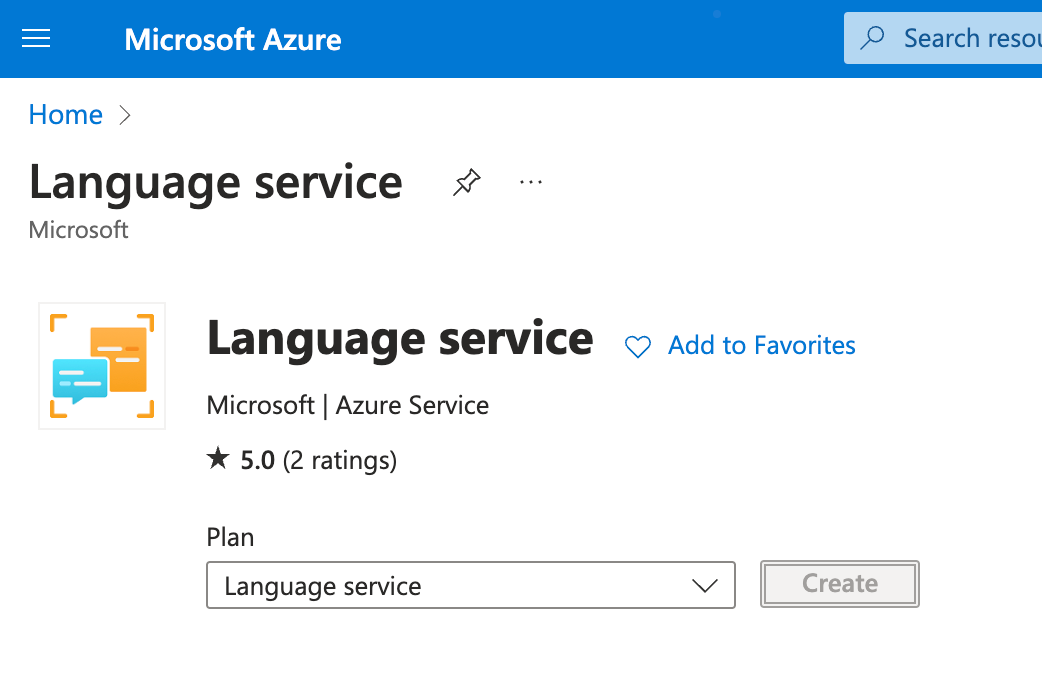
Select the feature for custom text classification and custom named entity recognition. Entity recognition will be able to recognize entities (i.e., the beverage "wine") from the text "I like to order a glass of wine."
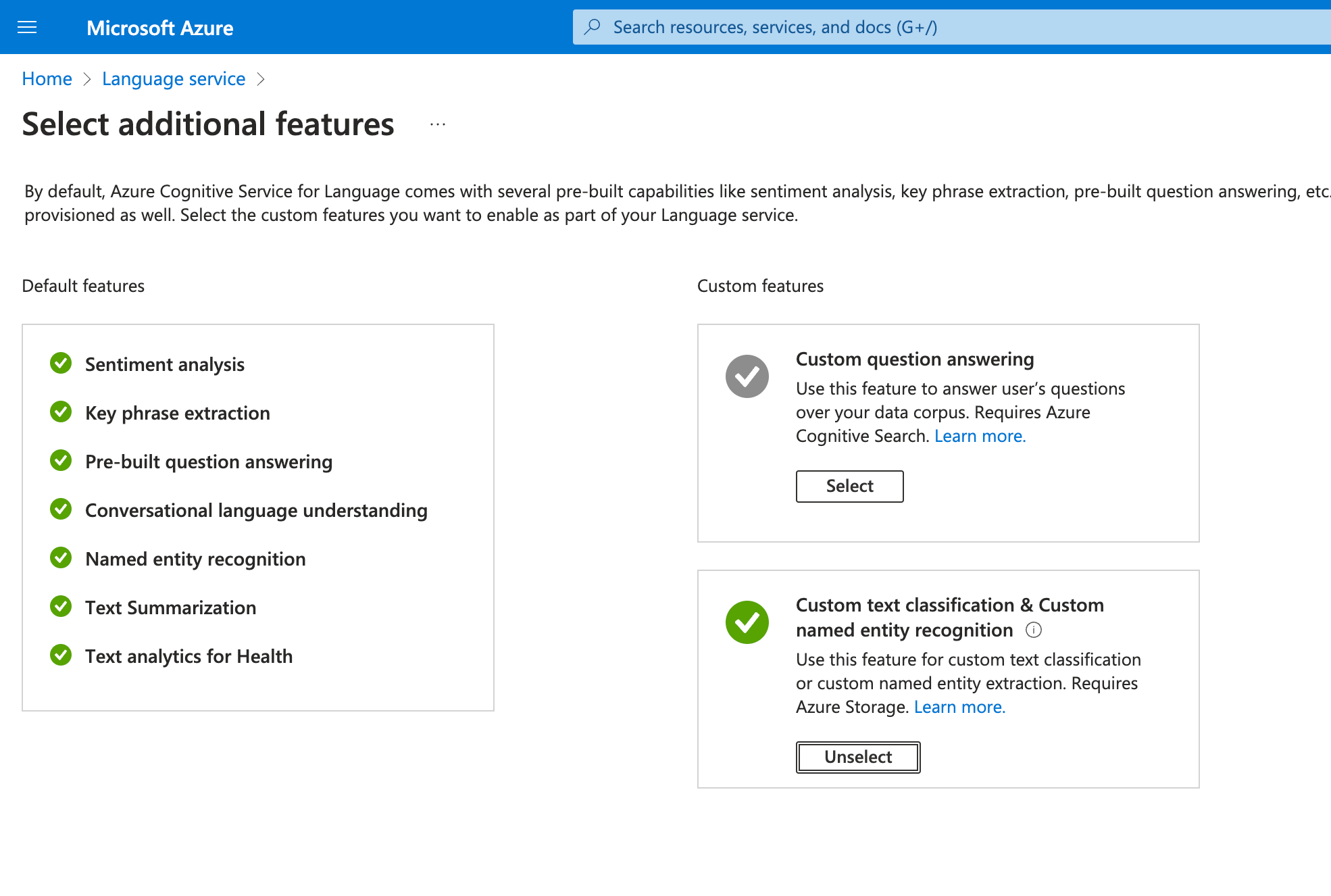
Deployment took around 5–10 minutes in my case. Once it is done, we can proceed and login to Microsoft Language Studio.
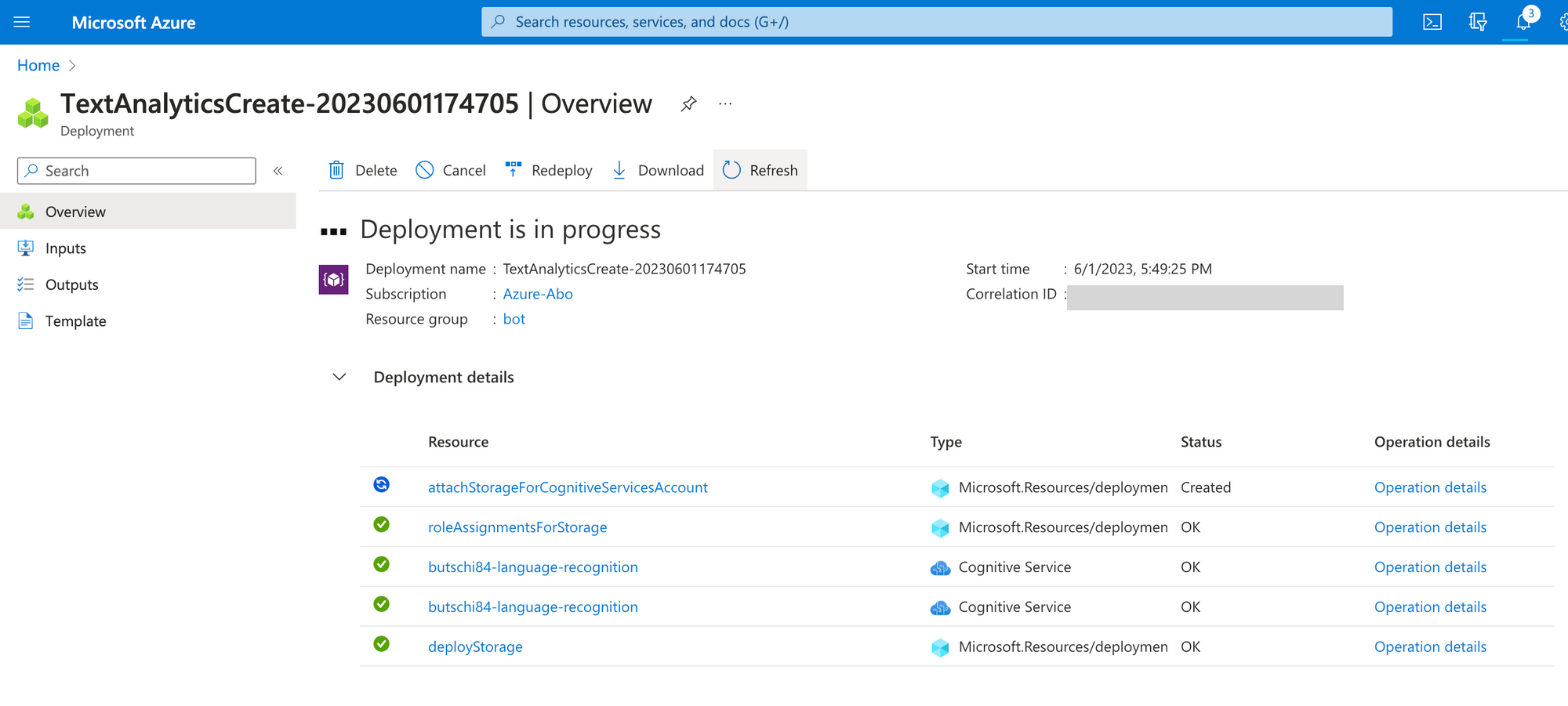
Training Language Model
Now you can login in Microsoft Language Studio and start to train a model: "Conversational Language understanding"
https://language.cognitive.azure.com/
There is also the possibility to import an example model so that you can have a look around and see how to configure everything in our own model later. You can find an example on this page. Search for "sample project".
In Part 4 of this blog series, we started a dialog design with a "order food" dialog and an "order beverages" dialog. In front of the two dialogs, there is an "intent dialog", that should decide whether the customer likes to order food or beverages. Recognizing this intent is the goal of our language model. You can configure your own model or import the one that I used for testing. My language model can be found in the Github repository.
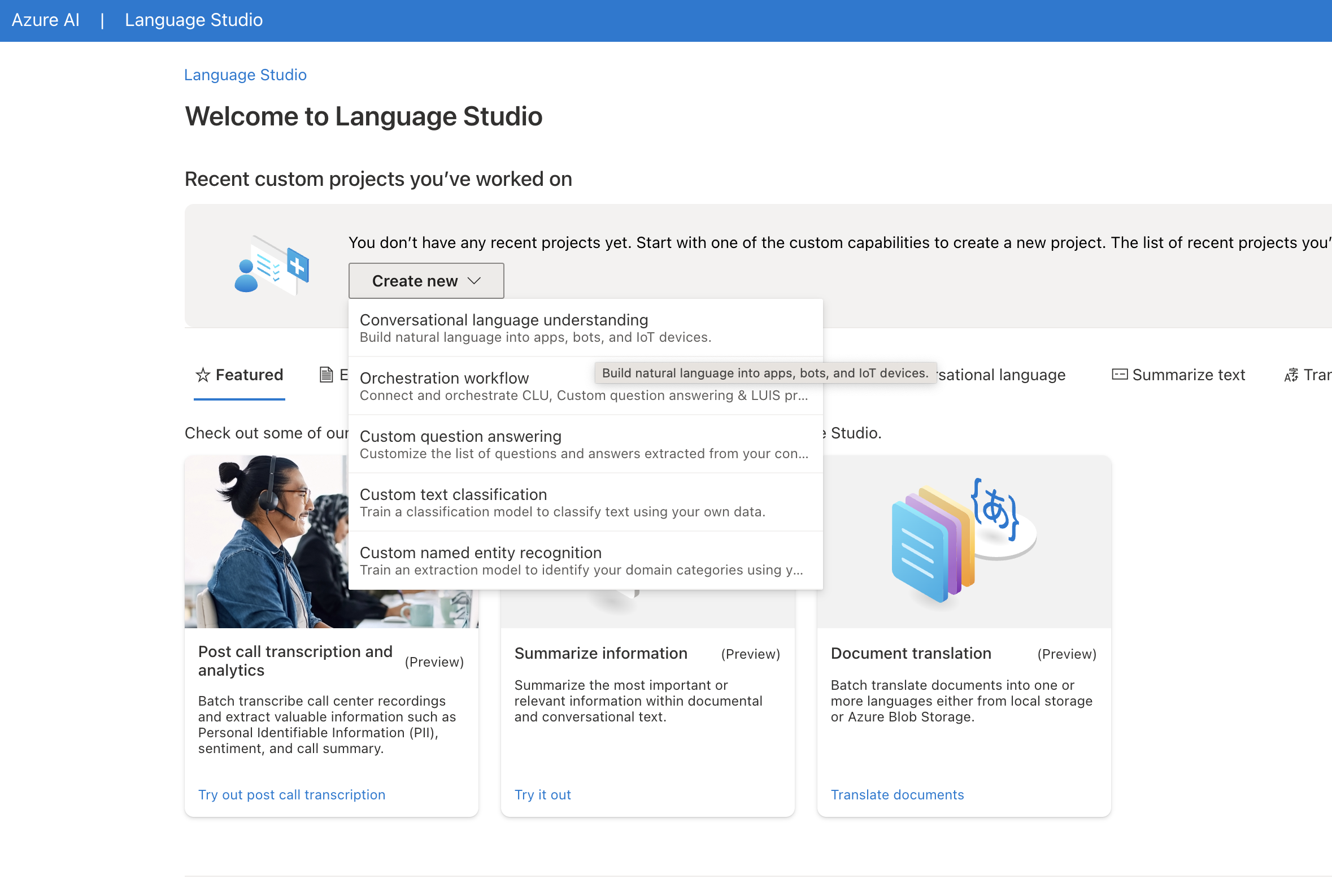
After the configuration of a language model, you should have a list of some intents (i.e. "order food" or "order beverages") with different utterances. I have just asked ChatGPT to generate me a list of different utterances, like:
- Could I have a cheeseburger with fries?
- I'd like to try your signature pasta dish.
- Can I get a side of garlic bread with my order?
- etc.
The labeling with "entities" is optional and could provide your bot with additional information about the user's intent. I did not play around too much with it at this point and like to keep it simple for now.
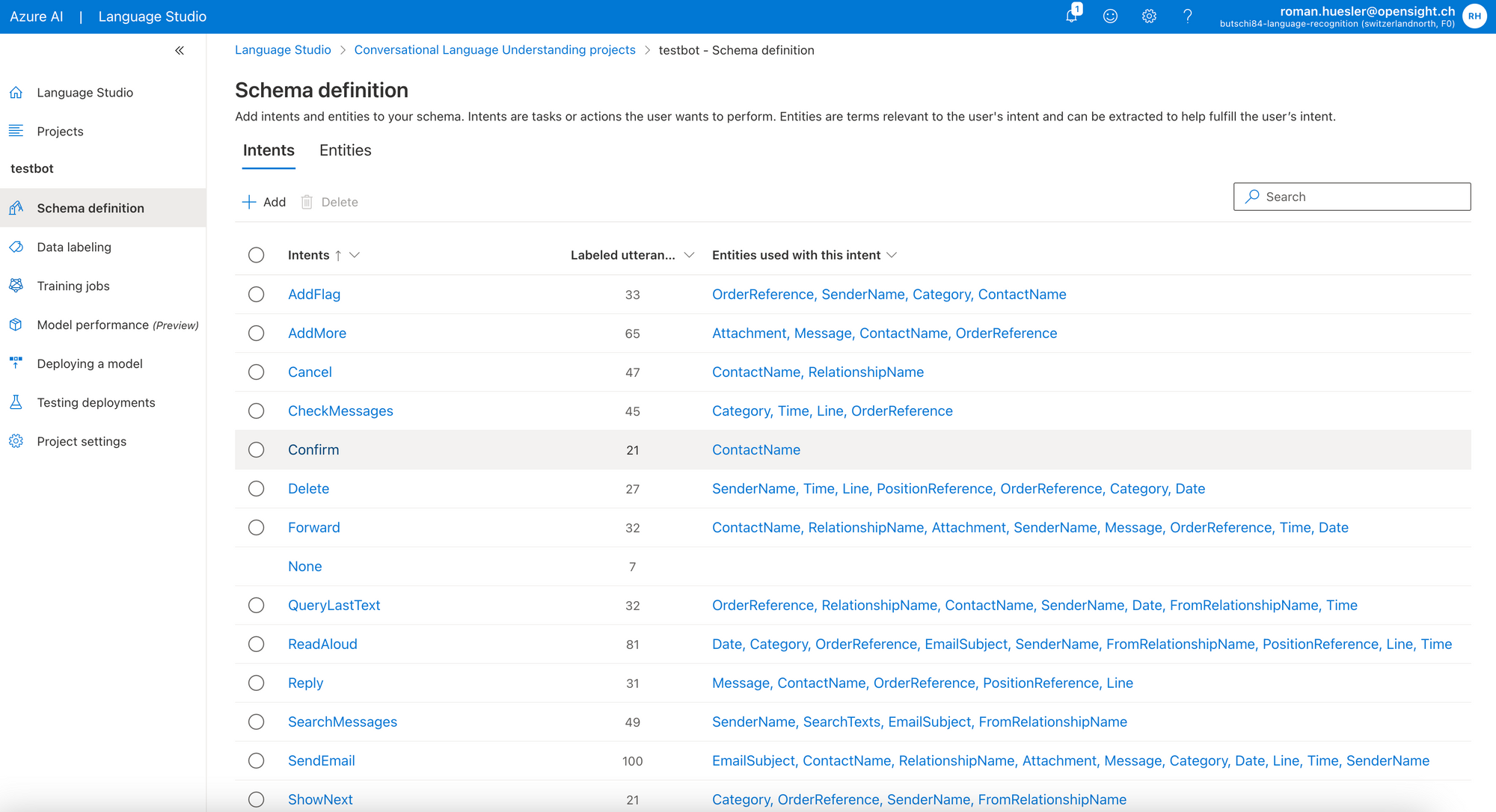
Once everything was configured, I needed to start a training job for the new language model. It took around 5 minutes to complete, and then the model was ready for deployment and testing.
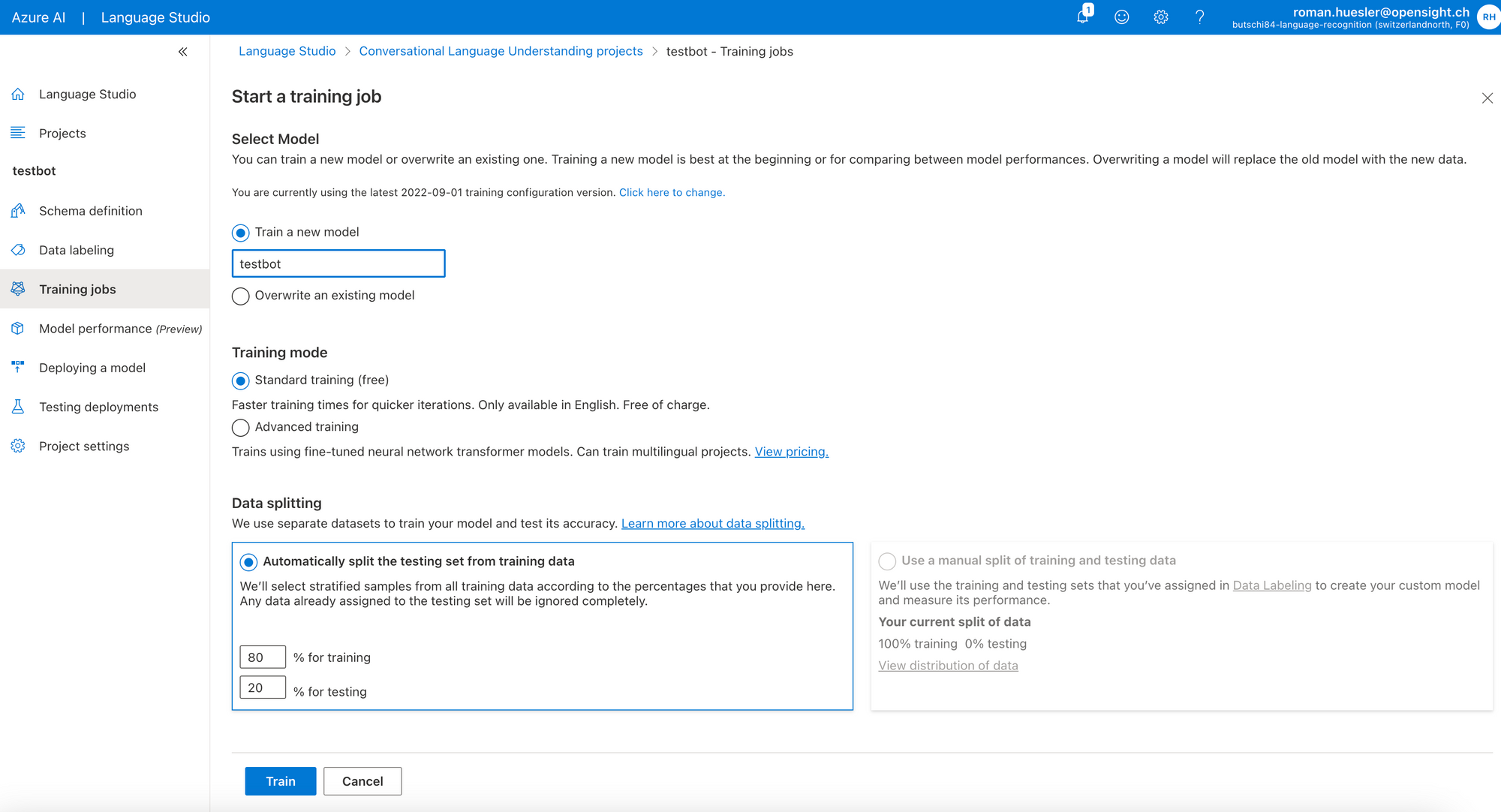
Create a new deployment in the "deploy a model" section.
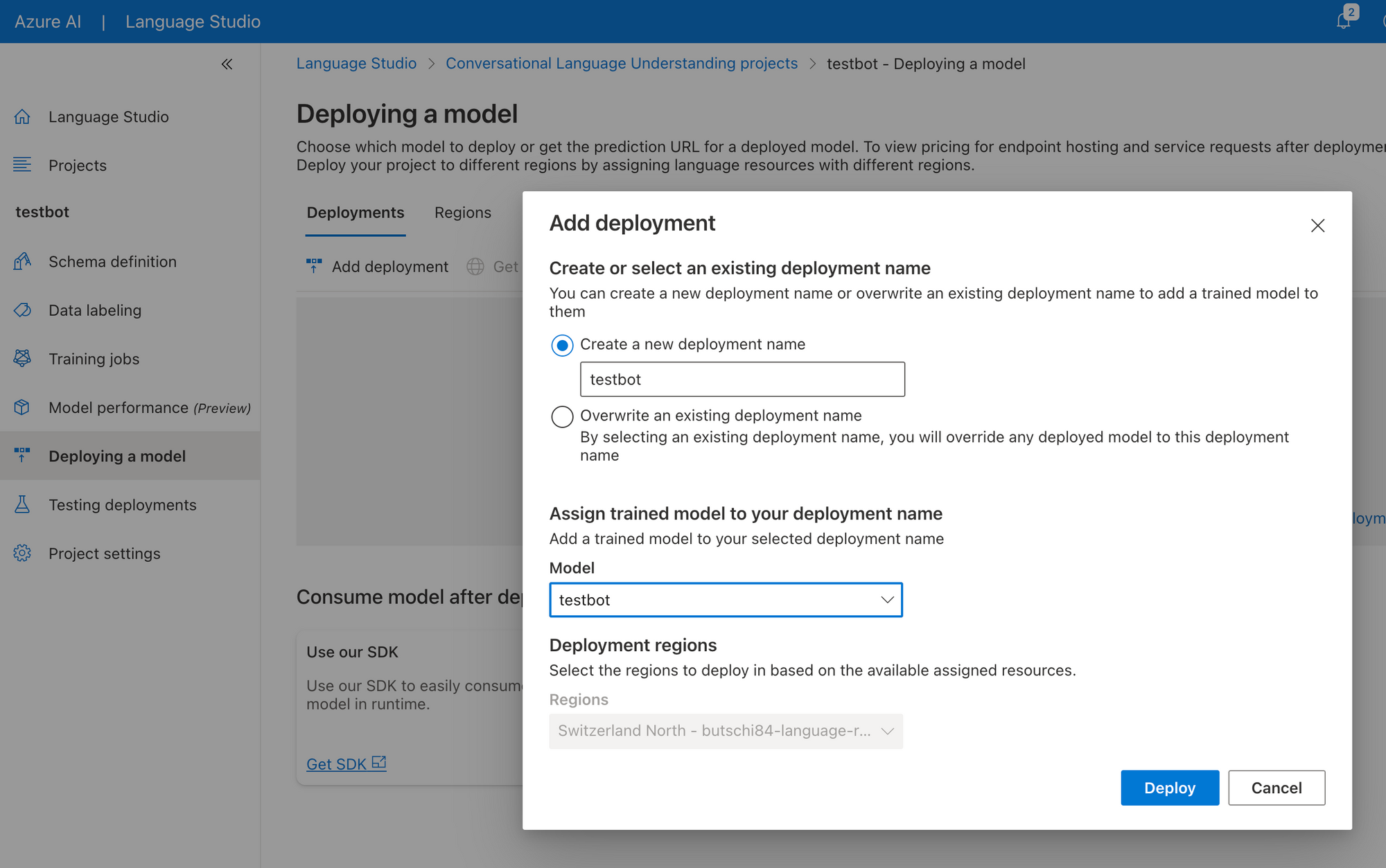
Now for the fun part. In "testing deployments" you can now start to test your language model. In our case you could enter something like: "What's the spiciest dish on your menu?" - and it should be able to derive the intent "order food". That's what we need for our chatbot. Now the language model is ready for implementation in the chatbot.
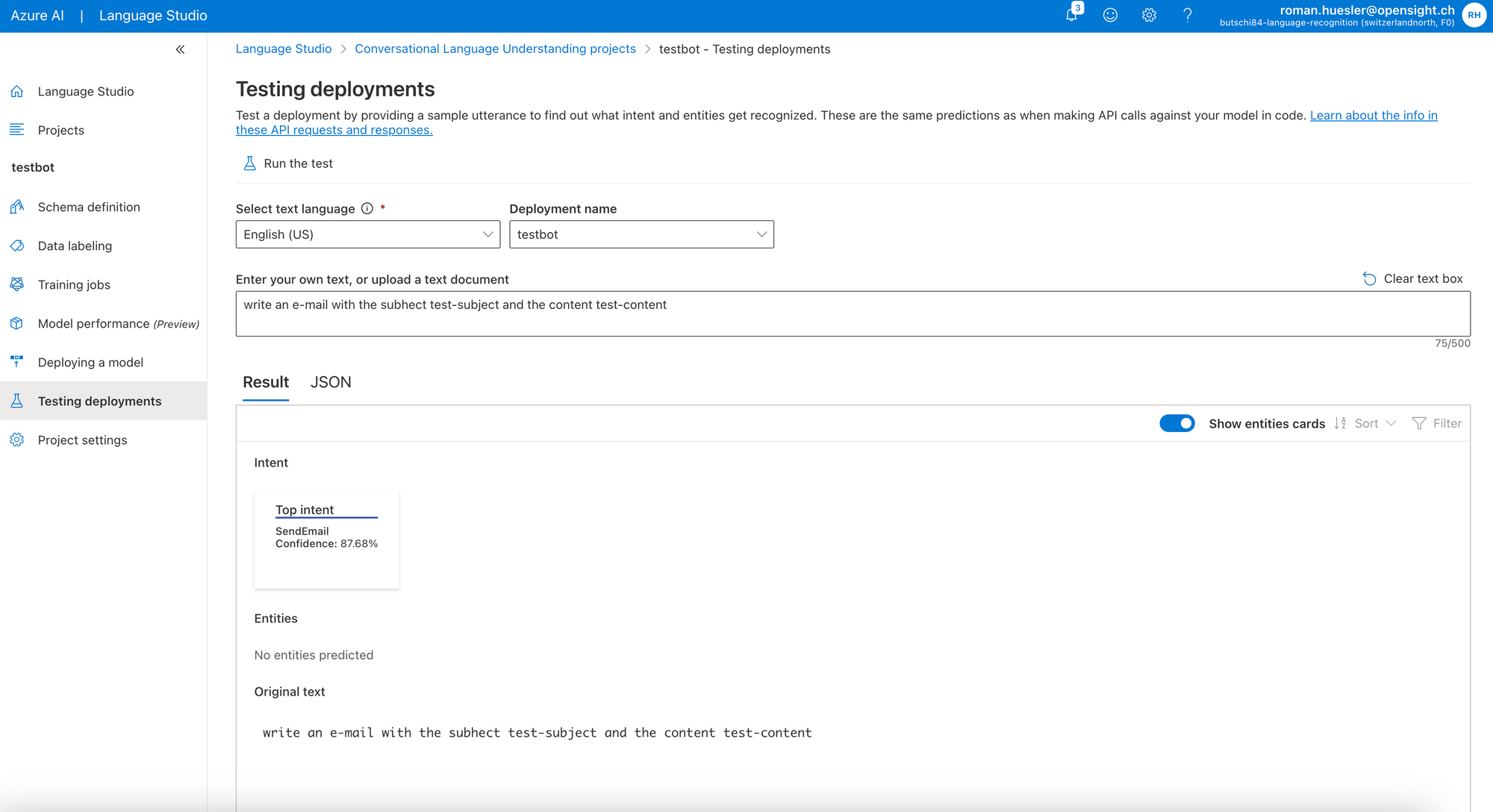
Bot Implementation
After deployment of the bot in Microsoft Language Studio, there is a button to "Get Prediction URL". It will show all the necessary information on how to send a POST request to the language model that we just created.
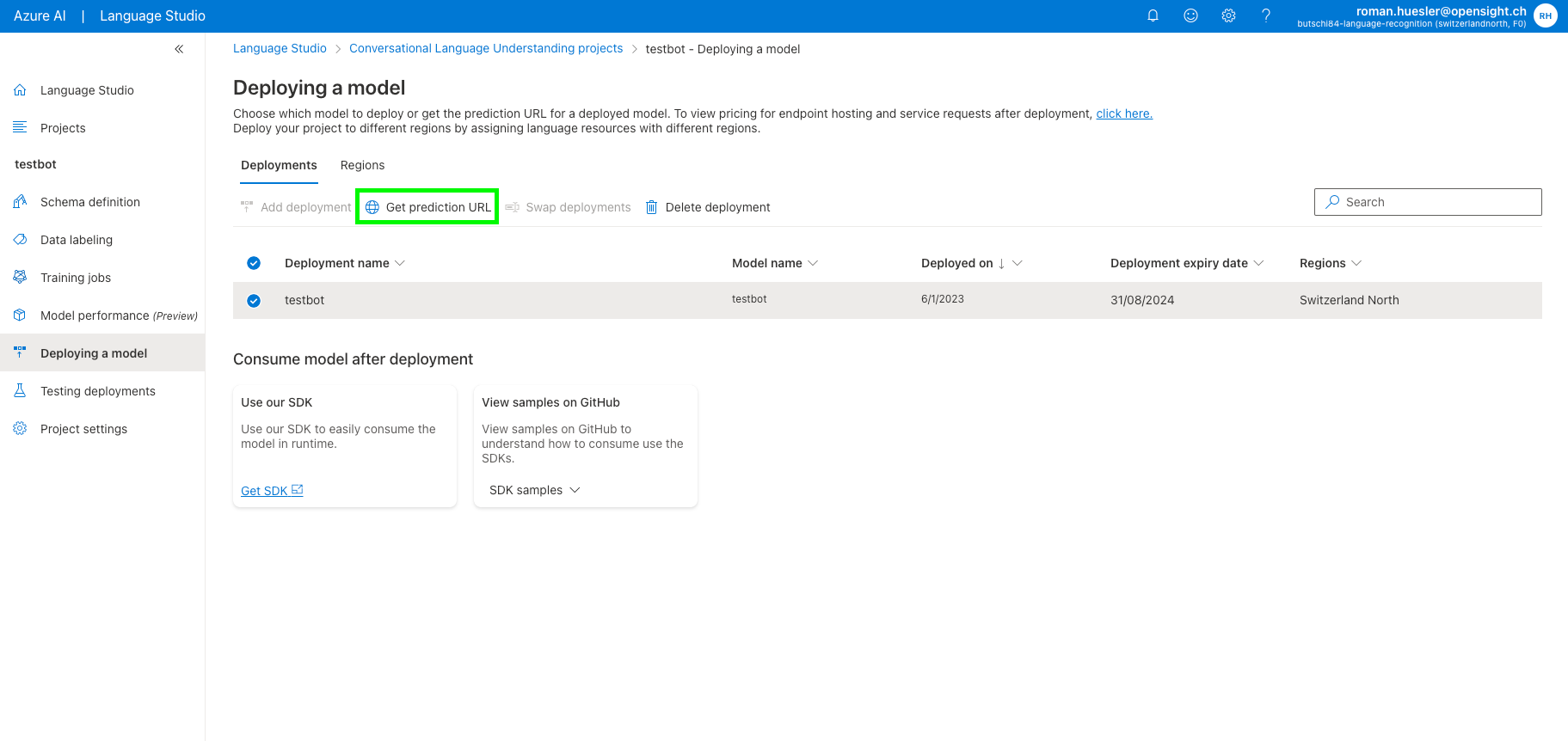
I quickly filled all the information into Postman and tried it successfully. So I think I will just use the Axios library in NodeJS to make a simple POST request in our "User Intent" part of the chatbot.
Let's install the Axios library, which will help us send a post request:
npm install --save axios
Then in intentDialog.js, I added an additional function "getUserIntentFromPrompt(prompt)" to query our new language model on Azure Conversational Language Understanding (CLU).
getUserIntentFromPrompt(prompt) {
return new Promise(async (resolve, reject) => {
const res = await axios.post('https://butschi84-language-recognition.cognitiveservices.azure.com/language/:analyze-conversations?api-version=2022-10-01-preview',
{
"kind": "Conversation",
"analysisInput": {
"conversationItem": {
"id": "roman",
"text": prompt,
"modality": "text",
"language": "EN",
"participantId": "roman"
}
},
"parameters": {
"projectName": "testbot",
"verbose": true,
"deploymentName": "testbot",
"stringIndexType": "TextElement_V8"
}
}, {
headers: {
'Ocp-Apim-Subscription-Key': '****',
'Apim-Request-Id': '*****',
'Content-Type': 'application/json'
}
});
resolve(res.data)
})
}
Also, I changed the prompt so that it is no longer a multiple choice prompt ("order food" or "order beverages") but instead a free text input: "I like to eat a big Caesar salad today".
Conclusion
Now when I open the chatbot in Microsoft Teams, I can send an arbitrary request and it will derive the intent "order food", "order beverages" and then start the relevant dialog. That's the next goal achieved: Test Azure Conversational Language Understanding (CLU)
You could also play around with Question and Answer (QnA) Databases in a similar manner with the language service.